yii2 框架的 orm 基础操作
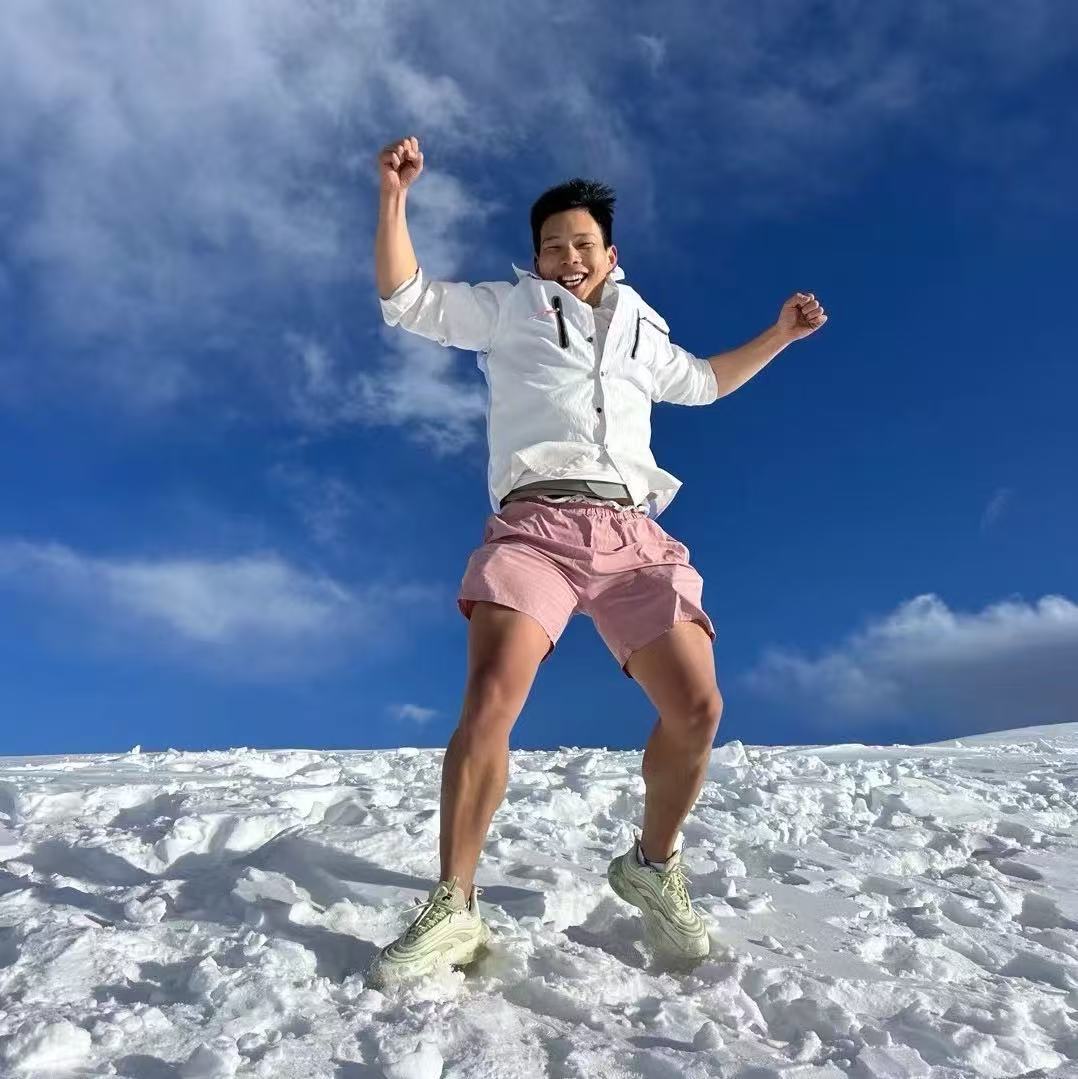
PS : CLASS STUDENT SCORE 默认为三张表的类
单条数据查询
1 | CLASS::findOne($id); |
多条数据查询
1 | CLASS::findAll(); |
单 where 条件
1 | CLASS::find()->where('id='.$id)->one(); |
多 where 条件
1 | //andWhere |
子查询
1 | SCORE::find('student_id')->where(['>','value',60])->andWhere( |
跨表查询
1 | CLASS::find()->alias(c)->where(['>',c.id,10]) |
复杂写法
1 | CLASS::find()->Where( |